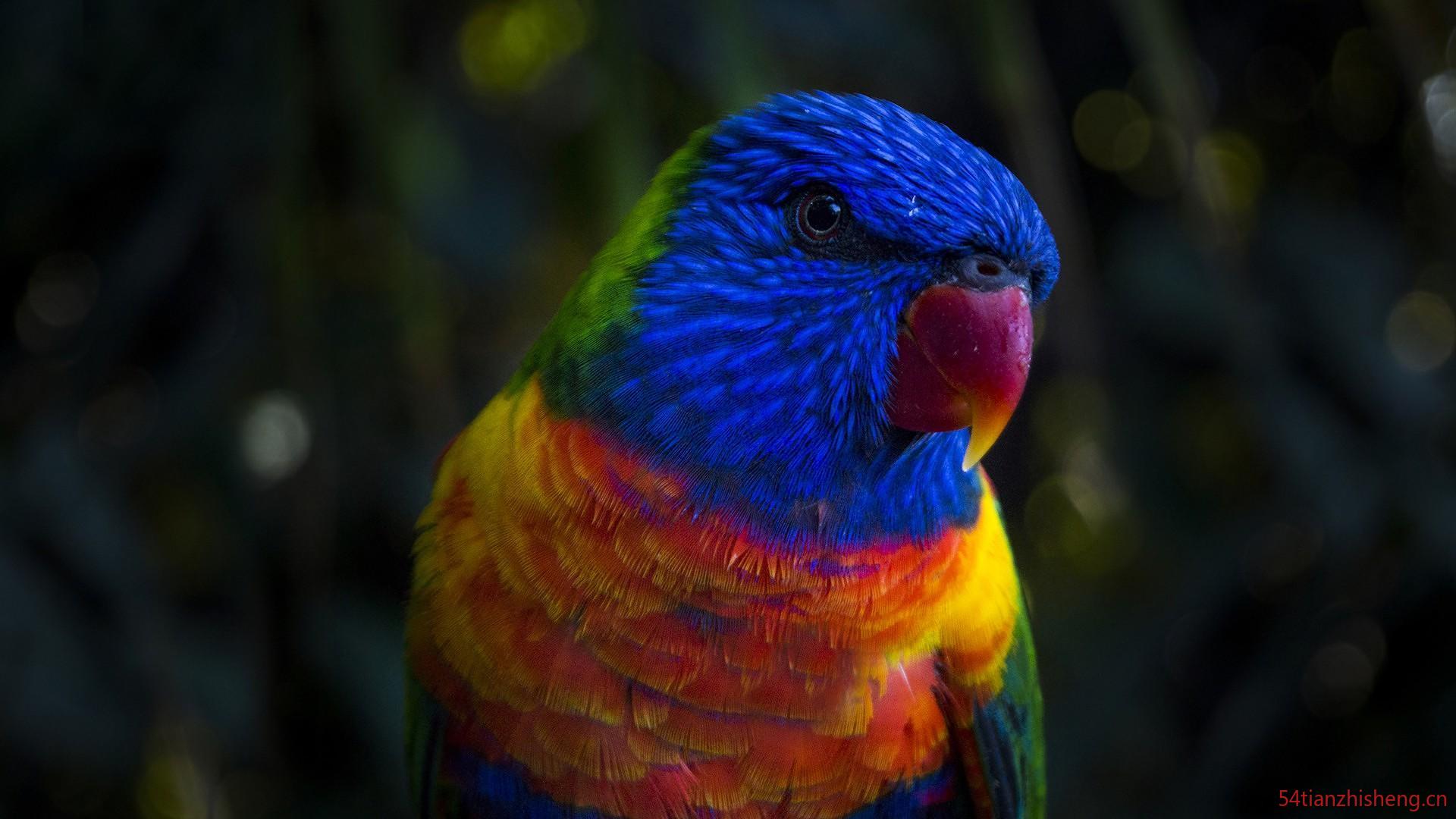
真乃神器也,再复杂的 Json 都能给你解析出来,非常方便的获取 JSON 的内容,很强大!
语法简介
JsonPath |
描述 |
$ |
根节点 |
@ |
当前节点 |
.or[] |
子节点 |
.. |
选择所有符合条件的节点 |
* |
所有节点 |
[] |
迭代器标示,如数组下标 |
[,] |
支持迭代器中做多选 |
[start:end:step] |
数组切片运算符 |
?() |
支持过滤操作 |
() |
支持表达式计算 |
JSON 值:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| { "store": { "book": [ { "category": "reference", "author": "Nigel Rees", "title": "Sayings of the Century", "price": 8.95 }, { "category": "fiction", "author": "Evelyn Waugh", "title": "Sword of Honour", "price": 12.99, "isbn": "0-553-21311-3" } ], "bicycle": { "color": "red", "price": 19.95 } } }
|
导包:import com.jayway.jsonpath.JsonPath
解析代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| String author = JsonPath.read(json, "$.store.book[0].author"); System.out.println("author\t"+author);
List<String> authors = JsonPath.read(json, "$.store.book[*].author"); System.out.println("authors\t"+authors);
List<Object> books = JsonPath.read(json, "$.store.book[?(@.category == 'reference')]"); System.out.println("books\t"+books);
List<Object> books2 = JsonPath.read(json, "$.store.book[?(@.category == 'reference' || @.price>10)]"); System.out.println("books2\t"+books2);
List<Object> books1 = JsonPath.read(json, "$.store.book[?(@.category == 'reference')].author"); System.out.println("books1\t"+books1);
List<Object> b1 = JsonPath.read(json, "$.store.book[?(@.price>10)]"); System.out.println("b1"+b1);
List<Object> b2 = JsonPath.read(json, "$.store.book[?(@.isbn)]"); System.out.println("b2"+b2);
List<Double> prices = JsonPath.read(json, "$..price"); System.out.println("prices"+prices);
List<Double> title = JsonPath.read(json, "$..title"); System.out.println("title"+title);
List<Double> book01 = JsonPath.read(json, "$..book[0,1]"); System.out.println("book01"+book01);
JsonPath path = JsonPath.compile("$.store.book[*]"); List<Object> b3 = path.read(json); System.out.println("path\t"+path+"\n"+b3);
|
用法比较简单,多使用几次就会使用了!文章主要参考网上!原谅很多天不跟博的我现在竟然这样水了这么一篇文章,哈哈!实在是忙!
